Programatically Creating Objects
+3
aheirich
sio2interactive
tbraun
7 posters
Page 1 of 2 • 1, 2
Programatically Creating Objects
I need to create some SIO2 objects programatically. It is a very simple object consisting of a single quad. I have found documentation on how to load multiple resources, and I guess it would be possible to add the quads to a resource and duplicate them as needed.
Is there a best practice to accomplish this? I have looked at all the examples and all of them load their resources from a sio2 file.
Thanks,
Tim
Is there a best practice to accomplish this? I have looked at all the examples and all of them load their resources from a sio2 file.
Thanks,
Tim
tbraun- Posts : 8
Join date : 2008-10-28
Re: Programatically Creating Objects
Im not sure what you are trying to achieve but... you can always load your "main" object from a .sio2 file and then use sio2ObjectDuplicate to create multiple instances of it...
How does that sound?
How does that sound?
Re: Programatically Creating Objects
I need to create a simple object at runtime. It is a single quad with a texture applied to it. The quad varies in size so a static resource will be difficult to create and use.
In other words, I will create the raw vertex data at runtime. How do I create a sio2 object to manage and render this data using the api.
Thanks,
Tim
In other words, I will create the raw vertex data at runtime. How do I create a sio2 object to manage and render this data using the api.
Thanks,
Tim
tbraun- Posts : 8
Join date : 2008-10-28
Re: Programatically Creating Objects
Unfortunately not. I just looked at the two functions and they don't seem to be able to handle what I need. I need more control over the object once it's created.
I would like to be able to take some raw vertex data/uv coordinates and create a SIO2Object with it. This way I can manage it the same way I manage all the other resources in the application. Ultimately, I could create my own VBO's and manage them manually, but it would be nice to be able to use the SIO2 API's throughout the application.
I don't think there is currently an official method to do this. I think the only current solution would be to mimic the loading methods used by the sio2 file loader. But instead of feeding data to the loader from a stream, feed it data I create at runtime.
Not sure if I'm being very clear on this one. For some reason, I'm finding it difficult to put words to the problem.
Thanks,
Tim
I would like to be able to take some raw vertex data/uv coordinates and create a SIO2Object with it. This way I can manage it the same way I manage all the other resources in the application. Ultimately, I could create my own VBO's and manage them manually, but it would be nice to be able to use the SIO2 API's throughout the application.
I don't think there is currently an official method to do this. I think the only current solution would be to mimic the loading methods used by the sio2 file loader. But instead of feeding data to the loader from a stream, feed it data I create at runtime.
Not sure if I'm being very clear on this one. For some reason, I'm finding it difficult to put words to the problem.
Thanks,
Tim
tbraun- Posts : 8
Join date : 2008-10-28
Re: Programatically Creating Objects
Ok then, for that you can check the "fileformat_specification.pdf" in the /Docs directory.
need to create physics objects programmatically
I want to second this request. I would also like to be able to create elements of my geometry at run time. Ideally this would not depend on having a Blender object but would allow us to define objects as GL vertex arrays with textures etc. But I still want to take advantage of all of sio2's neat features like rendering my scene for me. Some APIs to let us create and specify sio2 objects would be really helpful.
aheirich- Posts : 11
Join date : 2009-02-25
Re: Programatically Creating Objects
Well this is fairly easy... Put your vertex array in the SIO2object->buf, specify the offset in SIO2object->vbo_offset then create a SIO2vertexgroup for the indices and material info. Check the SIO2object loading mechanism for more info, but just to let you know technically speaking your a not tied to Blender in anyway.
Re: Programatically Creating Objects
would be cool to se some tutorial about this, I've been trying to make VBOs work and I must be forgetting something because it always crashes into gliDestroyContext() :S
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
I was trying to do the exact same thing about two weeks ago. Here is the code I used for a proof-of-concept, which was created by analyzing the SIO2object loading sequence:
As you can see, I'm using an existing object (default plane with 1x1 dimensions and no textures) exported by blender and I'm modifying it's contents (overwriting actually). This is just to make things simpler and I'm sure it will work fine if you create the object in runtime too, since pretty much everything in the object's structure is overwritten.
Also, there's a lot of hardcoded stuff in there, like the values in vbo_offset and the vertexes positions and colors and the triangles too. Obviously, for a real app, you will have to find a way to fill those values automatically. I think it's pretty clear what each values is and how it is calculated, but if you have any questions, I'll be happy to explain better.
Hope this helps,
André
- Code:
SIO2object *plane = ( SIO2object * )sio2ResourceGet( sio2->_SIO2resource, SIO2_OBJECT, "object/Plane" );
unsigned int boffset = 0, ioffset = 0;
vec3 v;
ind3 e;
col4 c;
// VBO_OFFSET
plane->vbo_offset[ SIO2_OBJECT_SIZE ] = 96;
plane->vbo_offset[ SIO2_OBJECT_VCOLOR ] = 72;
plane->vbo_offset[ SIO2_OBJECT_NORMALS ] = 0;
plane->vbo_offset[ SIO2_OBJECT_TEXUV0 ] = 0;
plane->vbo_offset[ SIO2_OBJECT_TEXUV1 ] = 0;
if( plane->vbo_offset[ SIO2_OBJECT_SIZE ] )
{ plane->buf = ( unsigned char * ) malloc( plane->vbo_offset[ SIO2_OBJECT_SIZE ] ); }
boffset = 0;
// VERT
v.x = 1; v.y = 1; v.z = 0;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
v.x = -1; v.y = 1; v.z = 0;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
v.x = -1; v.y = -1; v.z = 0;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
v.x = 1; v.y = -1; v.z = 0;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
v.x = 0; v.y = 0; v.z = 1;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
v.x = 0; v.y = -1.2; v.z = 0;
memcpy( &plane->buf[ boffset ], &v, 12 );
boffset += 12;
// VCOL
c.r = 0; c.g = 0; c.b = 0; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
c.r = 0; c.g = 255; c.b = 255; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
c.r = 255; c.g = 0; c.b = 255; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
c.r = 255; c.g = 255; c.b = 0; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
c.r = 255; c.g = 0; c.b = 0; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
c.r = 0; c.g = 0; c.b = 255; c.a = 255;
memcpy( &plane->buf[ boffset ], &c, 4 );
boffset += 4;
// N_VGROUP
plane->n_vgroup = 1;
if( plane->n_vgroup )
{ plane->_SIO2vertexgroup = ( SIO2vertexgroup ** ) malloc( plane->n_vgroup * sizeof( SIO2vertexgroup ) ); }
// VGROUP
plane->_SIO2vertexgroup[ 0 ] = sio2VertexGroupInit( "null" );
// N_IND
plane->_SIO2vertexgroup[ 0 ]->n_ind = 15;
if( plane->_SIO2vertexgroup[ 0 ]->n_ind )
{ plane->_SIO2vertexgroup[ 0 ]->ind = ( unsigned short * ) malloc( plane->_SIO2vertexgroup[ 0 ]->n_ind << 1 ); }
ioffset = 0;
// IND
e.x = 0; e.y = 1; e.z = 4;
memcpy( &plane->_SIO2vertexgroup[ 0 ]->ind[ ioffset ], &e, 6 );
ioffset += 3;
e.x = 1; e.y = 2; e.z = 4;
memcpy( &plane->_SIO2vertexgroup[ 0 ]->ind[ ioffset ], &e, 6 );
ioffset += 3;
e.x = 2; e.y = 3; e.z = 4;
memcpy( &plane->_SIO2vertexgroup[ 0 ]->ind[ ioffset ], &e, 6 );
ioffset += 3;
e.x = 0; e.y = 4; e.z = 3;
memcpy( &plane->_SIO2vertexgroup[ 0 ]->ind[ ioffset ], &e, 6 );
ioffset += 3;
e.x = 2; e.y = 5; e.z = 3;
memcpy( &plane->_SIO2vertexgroup[ 0 ]->ind[ ioffset ], &e, 6 );
ioffset += 3;
sio2ResourceBindMaterial(sio2->_SIO2resource, plane);
sio2ResourceBindInstance(sio2->_SIO2resource, plane);
sio2TransformBindMatrix(plane->_SIO2transform);
sio2ObjectGenId(plane);
for (int j = 0; j != plane->n_vgroup; j++)
sio2VertexGroupGenId( plane->_SIO2vertexgroup[ j ] );
sio2ObjectReset();
sio2ResourceResetState();
As you can see, I'm using an existing object (default plane with 1x1 dimensions and no textures) exported by blender and I'm modifying it's contents (overwriting actually). This is just to make things simpler and I'm sure it will work fine if you create the object in runtime too, since pretty much everything in the object's structure is overwritten.
Also, there's a lot of hardcoded stuff in there, like the values in vbo_offset and the vertexes positions and colors and the triangles too. Obviously, for a real app, you will have to find a way to fill those values automatically. I think it's pretty clear what each values is and how it is calculated, but if you have any questions, I'll be happy to explain better.
Hope this helps,
André
Re: Programatically Creating Objects
Look like a start 
However for:
sio2ResourceBindMaterial(sio2->_SIO2resource, plane);
>> Its ok, but you should assign at least one material to the vertex group, instead this have no effect.
sio2ResourceBindInstance(sio2->_SIO2resource, plane);
>> Instance are for child object, you are creating a "master", this is also obsolete.
And also this is in example the output of for a plane with vertex color:
Maybe you should double check your code...
And for the VBO_OFFSET make sure that you are following that sequence:
Cheers,

However for:
sio2ResourceBindMaterial(sio2->_SIO2resource, plane);
>> Its ok, but you should assign at least one material to the vertex group, instead this have no effect.
sio2ResourceBindInstance(sio2->_SIO2resource, plane);
>> Instance are for child object, you are creating a "master", this is also obsolete.
And also this is in example the output of for a plane with vertex color:
- Code:
object( "object/Plane" )
{
loc( 0 0 0 )
rad( 1.414 )
bounds( 4 )
dim( 1 1 0 )
vbo_offset( 64 48 0 0 0 )
vert( 1 1 0 )
vert( -1 1 0 )
vert( -1 -1 0 )
vert( 1 -1 0 )
vcol( 217 114 114 )
vcol( 95 229 148 )
vcol( 174 230 62 )
vcol( 129 132 222 )
n_vgroup( 1 )
vgroup( "null" )
n_ind( 6 )
ind( 0 1 2 )
ind( 0 2 3 )
}
Maybe you should double check your code...
And for the VBO_OFFSET make sure that you are following that sequence:
- Code:
typedef enum
{
SIO2_OBJECT_SIZE = 0,
SIO2_OBJECT_VCOLOR,
SIO2_OBJECT_NORMALS,
SIO2_OBJECT_TEXUV0,
SIO2_OBJECT_TEXUV1,
SIO2_OBJECT_NVBO_OFFSET
} SIO2_OBJECT_VBO_OFFSET;
Cheers,
Re: Programatically Creating Objects
Thanks for the tips guys, but I needed to draw my mesh class instead of the sio2object one. All that I needed was a clear working VBO example, when I found it, it was pretty quick to make it work with my mesh class ^^
found it here, I hope it helps someone
Now I am only having one small problem, if I use sio2FontPrint() it goes crazy and mixes everything. I guess that it will happen also when using widgets. Why is this happening? :S
I attach the code, I can also attach a screenshot with what it does when goes crazy.
I know that for the example right now I am not using glEnter...3D, sio2WindowEnter...2D, but that still draws on screen, the problem is when using sio2FontPrint() it does not work well. any idea?
found it here, I hope it helps someone

Now I am only having one small problem, if I use sio2FontPrint() it goes crazy and mixes everything. I guess that it will happen also when using widgets. Why is this happening? :S
I attach the code, I can also attach a screenshot with what it does when goes crazy.
- Code:
void templateRender( void )
{
if(!first)
{
SIO2stream *_SIO2stream;
_SIO2stream = sio2StreamInit("cargador");
_SIO2stream = sio2StreamOpen("baby.obj",1);
m=LoadObj(_SIO2stream);
_SIO2stream = sio2StreamClose(_SIO2stream);
/*vbo stuff*/
glGenBuffers(1, &meshVBO);
glBindBuffer(GL_ARRAY_BUFFER, meshVBO);
const GLsizeiptr stride = 3 * sizeof(float) + 4 * sizeof(char);
glBufferData(GL_ARRAY_BUFFER, m.numberOfVertices*sizeof(vertex), 0, GL_STATIC_DRAW);
glBufferSubData(GL_ARRAY_BUFFER, 0, m.numberOfVertices*sizeof(vertex), m.vertexArray); // start at index 0, to length of vertex_size
glVertexPointer(3, GL_FLOAT, stride, (GLvoid*)((char*)NULL));
glColorPointer(4, GL_UNSIGNED_BYTE, stride, (GLvoid*)((char*)NULL+4*sizeof(char)));
glGenBuffers(1, &meshIBO);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, meshIBO);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, m.numberOfTriangles*sizeof(triangle), m.triangleArray, GL_STATIC_DRAW);
initFont();
first=1;
}
//Empiezan VBOs
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrthof(-1.5f, 1.5f, -2.5f, 2.5f, -10.5f, 10.5f);
glMatrixMode(GL_MODELVIEW);
//sio2WindowEnterLandscape3D();
//{
glRotatef(0.1f, 0.3f, 0.5f, 0.0f);
glClearColor(0.5f, 0.5f, 0.5f, 1.0f);
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
// Activate the VBOs to draw
glBindBuffer(GL_ARRAY_BUFFER, meshVBO);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, meshIBO);
// This could actually be moved into the setup since we never disable it
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
// This is the actual draw command
glDrawElements(GL_TRIANGLES, m.numberOfTriangles*3, GL_UNSIGNED_SHORT, (GLvoid*)((char*)NULL));
printf("\nFPS: %.f", sio2->_SIO2window->fps);
//}
//sio2WindowLeaveLandscape3D();
//final VBOs
//glMatrixMode( GL_MODELVIEW );
//glLoadIdentity();
//glClear( GL_DEPTH_BUFFER_BIT | GL_COLOR_BUFFER_BIT );
/*vec2 pos;
sio2WindowEnter2D( sio2->_SIO2window, 0.0f, 1.0f );
{
sio2FontPrint(fontCalibri, &pos, "FPS: %.f", sio2->_SIO2window->fps);
}
// Leave the 2D mode.
sio2WindowLeave2D();*/
}
I know that for the example right now I am not using glEnter...3D, sio2WindowEnter...2D, but that still draws on screen, the problem is when using sio2FontPrint() it does not work well. any idea?
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
Call:
sio2FontReset()
sio2ObjectReset()
sio2MaterialReset()
at the end of the block above...
sio2FontReset()
sio2ObjectReset()
sio2MaterialReset()
at the end of the block above...
Re: Programatically Creating Objects
oops, I forgot about the reset thing for the fonts
but the problem persists when drawing fonts, maybe is because it "mixes" text and mesh VBOs... I mean, if that's possible at all... :S ...vbos are new to me
I attach an image which pretty much explains what happens, the first one is without sio2fontprint call, the other one is what happens when I use it. The code remains the same but with all tree resets.
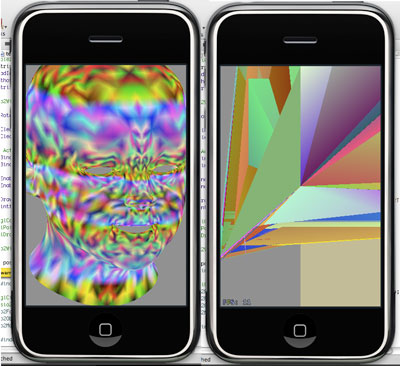
btw, vbo rocks! yesterday I tried to load up a 31K triangles obj and fps were around 40, well, no lights and almost nothing, just on that example, I guess that when I include this into the source it won't be that much but its perfect for what I wanted

I attach an image which pretty much explains what happens, the first one is without sio2fontprint call, the other one is what happens when I use it. The code remains the same but with all tree resets.
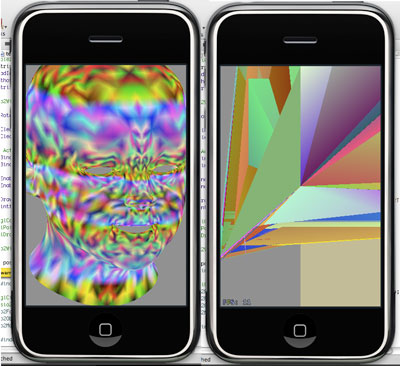
btw, vbo rocks! yesterday I tried to load up a 31K triangles obj and fps were around 40, well, no lights and almost nothing, just on that example, I guess that when I include this into the source it won't be that much but its perfect for what I wanted

exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
31K triangles obj and fps were around 40 ??
how did you do this ? the version 1.3.3 ?
and did you have modified some sio2 source code ?
how did you do this ? the version 1.3.3 ?
and did you have modified some sio2 source code ?
sw- Posts : 73
Join date : 2008-10-12
Re: Programatically Creating Objects
yes but I that fps its a bit tricky like I said before so at this point this does not mean much...
let me explain.
In my app I am only going to have 1 mesh so I tried with a good one. That mesh has 31040 tris.
for this vbo example I was only using vertex data and vertex colors, no normals, no lights yet which will make its performance drop heavily when set up.
both vertex and vcol are set up as GL_STATIC (in my app I will use vertex as DYNAMIC)
I am not using any physics either
at this point in the example I am only using templateRender function, no resources loaded, texture font builded, nothing more. I am not using any sio2function at this point. (in the example, in the app source I am using only sio2UniqueColor and a couple more only for now...) so I guess that its release independant. I am drawing on screen my obj loaded so no sio2object used either.
the fps count went from 30 to 50 for a minute then it stayed at about 40 in the simulator in debug mode
I have to say that, as I am unable at this point to print fps in the viewport with sio2fontprint() i was printing them with printf at console.
let me explain.
In my app I am only going to have 1 mesh so I tried with a good one. That mesh has 31040 tris.
for this vbo example I was only using vertex data and vertex colors, no normals, no lights yet which will make its performance drop heavily when set up.
both vertex and vcol are set up as GL_STATIC (in my app I will use vertex as DYNAMIC)
I am not using any physics either
at this point in the example I am only using templateRender function, no resources loaded, texture font builded, nothing more. I am not using any sio2function at this point. (in the example, in the app source I am using only sio2UniqueColor and a couple more only for now...) so I guess that its release independant. I am drawing on screen my obj loaded so no sio2object used either.
the fps count went from 30 to 50 for a minute then it stayed at about 40 in the simulator in debug mode
I have to say that, as I am unable at this point to print fps in the viewport with sio2fontprint() i was printing them with printf at console.
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
the performance on the simulator are TOTALLY different than the device really... its about 1/3 (2nd gen) to 1/5 (1st gen) of the one you get in the sim.
Re: Programatically Creating Objects
wow, didn't know that it was different. on the game I did with sio2 its equal or really close because we didn't noticed, I will try the same mesh on the device when I have some time. anyways I said not to take those values much seriously since were not on a "real" case scenario.
sio2, any idea why is messing vbos when rendering text? :S
sio2, any idea why is messing vbos when rendering text? :S
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
2 possibilities you didn't turn off so clientstates or your vbo have an offset that push the memory block or corrupt it... This more a gl issue than a sio2 one...
Re: Programatically Creating Objects
And by the way which game you do with sio2 I nver receive any mail from you... Hope you are respecting the eula hahaha
Re: Programatically Creating Objects
sio2interactive wrote:And by the way which game you do with sio2 I nver receive any mail from you... Hope you are respecting the eula hahaha
nonono, please don't get me wrong... I talked to you about the game. Its my degree project, its not a commercial game. Its not going to be released on the appstore, no one is going to have this game, just me and a classmate (since we both worked on it). I will send you the email as soon as I finish the thesis. Which I expect to be in a couple weeks.
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
simulator .hehe 
you will meet a big problem when you turn to device.


you will meet a big problem when you turn to device.


sw- Posts : 73
Join date : 2008-10-12
Re: Programatically Creating Objects
hehe, sure, thats why I was not taking these values much seriouslysw wrote:simulator .hehe
you will meet a big problem when you turn to device.![]()

tried everything, enabling/disabling client states, commented the interleaved arrays part of the code, created several different vbos and everything works fine and draws correctly, in its place, unless i use sio2fontprint .... this is reeeeaaaally weird :S
exavi- Posts : 37
Join date : 2008-10-21
Re: Programatically Creating Objects
Check the code of sio2FontPrint, that might give you an idea where the problem comes from...
Re: Programatically Creating Objects
wow, my mistake. I did not noticed that I was calling pointers (glVertexPointer(), glColorPointer....) when initializing instead of when rendering :S that will work ok for any render element created at initialization, but in this case sio2FontBuild was not initialized at the same place so that was why was doing weird stuff..
the fix was just calling pointers right before rendering them (which is the correct way to do so, but as I was using this example, I thought that it could not be that important)
now everything works perfect.
I paste here the code, hope this helps someone.
the fix was just calling pointers right before rendering them (which is the correct way to do so, but as I was using this example, I thought that it could not be that important)
now everything works perfect.
I paste here the code, hope this helps someone.
- Code:
const GLsizeiptr stride = 3 * sizeof(float) + 4 * sizeof(char);
void templateRender( void )
{
if(!first)
{
initFont();
SIO2stream *_SIO2stream;
_SIO2stream = sio2StreamInit("cargador");
_SIO2stream = sio2StreamOpen("baby.obj",1);
m=LoadObj(_SIO2stream);
_SIO2stream = sio2StreamClose(_SIO2stream);
/*vbo stuff*/
glGenBuffers(1, &meshVBO);
glBindBuffer(GL_ARRAY_BUFFER, meshVBO);
// allocate enough space for the VBO
glBufferData(GL_ARRAY_BUFFER, m.numberOfVertices*stride, 0, GL_STATIC_DRAW);
glBufferSubData(GL_ARRAY_BUFFER, 0, m.numberOfVertices*sizeof(vertex), m.vertexArray);
// create index buffer
glGenBuffers(1, &meshIBO);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, meshIBO);
glBufferData(GL_ELEMENT_ARRAY_BUFFER, m.numberOfTriangles*sizeof(triangle), m.triangleArray, GL_STATIC_DRAW);
first=1;
}
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrthof(-1.5f, 1.5f, -2.5f, 2.5f, -10.5f, 10.5f);
glMatrixMode(GL_MODELVIEW);
//sio2WindowEnterLandscape3D();
{
glRotatef(0.1f, 0.3f, 0.5f, 0.0f);
glClearColor(0.5f, 0.5f, 0.5f, 1.0f);
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
// Activate the VBOs to draw
glBindBuffer(GL_ARRAY_BUFFER, meshVBO);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, meshIBO);
glVertexPointer(3, GL_FLOAT, 0, (GLvoid*)((char*)NULL));
glColorPointer(4, GL_UNSIGNED_BYTE, stride, (GLvoid*)((char*)NULL+4*sizeof(char)));
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
glDrawElements(GL_TRIANGLES, m.numberOfTriangles*3, GL_UNSIGNED_SHORT, (GLvoid*)((char*)NULL));
glBindBuffer(GL_ARRAY_BUFFER, 0);
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER,0);
if( glIsEnabled( GL_COLOR_ARRAY ) )
{ glDisableClientState( GL_COLOR_ARRAY ); }
if( glIsEnabled( GL_NORMAL_ARRAY ) )
{ glDisableClientState( GL_NORMAL_ARRAY ); }
/*glColor4ub(255, 255, 255, 255);
glPointSize(3.0f);
glDrawArrays(GL_POINTS, 0, m.numberOfVertices);*/
}
//sio2WindowLeaveLandscape3D();
vec2 pos;
sio2WindowEnter2D( sio2->_SIO2window, 0.0f, 1.0f );
{
sio2FontPrint(fontCalibri, &pos, "FPS: %.f", sio2->_SIO2window->fps);
sio2FontReset();
sio2ObjectReset();
sio2MaterialReset();
}
sio2WindowLeave2D();
}
exavi- Posts : 37
Join date : 2008-10-21
Page 1 of 2 • 1, 2

» duplicating objects at runtime and changing textures on the duplicated objects
» Programatically applying a texture
» Attached objects?
» Duplicating objects
» Static Objects
» Programatically applying a texture
» Attached objects?
» Duplicating objects
» Static Objects
Permissions in this forum:
You cannot reply to topics in this forum